- 2d Parity Check Program Code
- 2d Parity Check Program Compatibility
- 2d Parity Check Program Cal
- 2d Parity Check Program Can
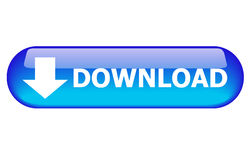
In this tutorial, we are going to learn how to find parity of a number efficiently in C++. Here we will learn about the parity of a number, algorithm to find the parity of a number efficiently, and after that, we will see the C++ program for the same.
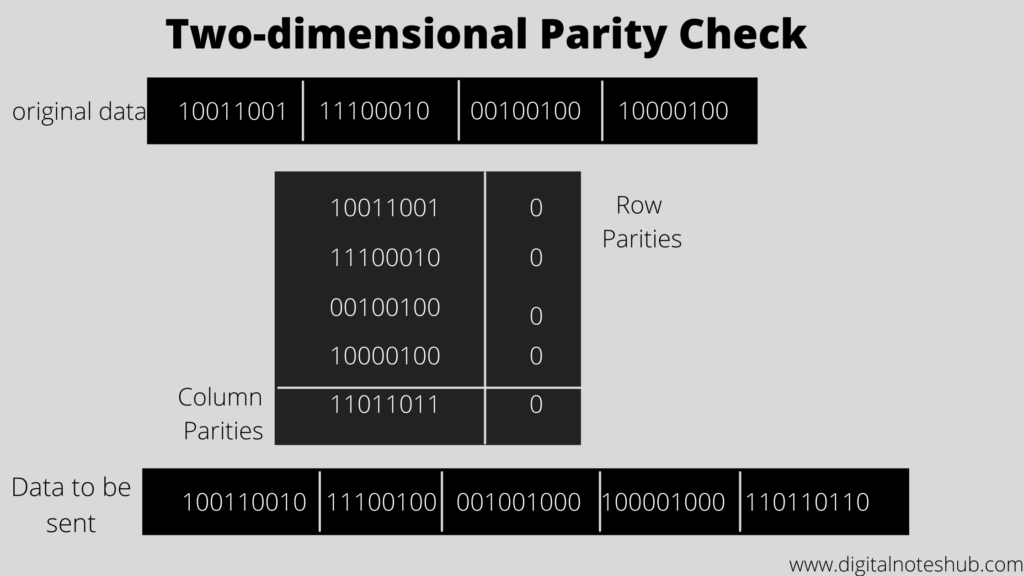
This program demonstrates 2d parity check when data is sent through physical layer - srishab55/2d-Parity-Check. If the rightmost bit is 1, then n will have odd parity and if it is 0 then n will have even parity. We check the odd or even by using bitwise AND operator. If (b & 1) is true means odd else even. C program to find parity of a number efficiently. So, here is the C implementation of the above algorithm. A Web Development & Designing and programming code. Learn Wordpress tips and hacks From Videos and code in Description. Languages used are C, C, C#, PHP, Mysql for developing Websites, Computer Network Programs, Android, Data Structure Programs, Object-Oriented Programming and more Google me: 'Sahil Gulati Surat'. Parity byte or parity word. The simplest checksum algorithm is the so-called longitudinal parity check, which breaks the data into 'words' with a fixed number n of bits, and then computes the exclusive or (XOR) of all those words. The result is appended to the message as an extra word.
Parity of a number
Parity of a number is a term used to tell that the total number of set bits(1’s) in its binary representation is even or odd. It is of two types:-
1. Even Parity: If the total number of set bits (1’s) is even, then that number has even parity.
2. Odd Parity: If the total number of set bits (1’s) is odd, then that number has odd parity.
For example:-
12 has even parity because it has an even number of set bits in its binary representation i.e ‘1100’.
14 has odd parity because it has an odd number of set bits in its binary representation i.e ‘1110’.
Efficient Algorithm to find parity of a number
The most efficient way of finding the parity of a number is by using XOR and shifting operator as shown below.
b = n ^ (n >> 1);
b = b ^ (b >> 2);
b = b ^ (b >> 4);
b = b ^ (b >> 8);
b = b ^ (b >> 16);
2d Parity Check Program Code
In the above operations, we use XOR and left shift operator. We are shifting double bits of the previous operation. And taking the XOR with that number. We know that 0 XOR 1 or 1 XOR 0 is 1, otherwise 0. So when we divide the binary representation of a number into two equal halves by length & we do XOR between them, all different pairs of bits result into set bits in the result number i.e “b” here.
Now, after the above operations b contain the rightmost bit of b and represent the parity of n. If the rightmost bit is 1, then n will have odd parity and if it is 0 then n will have even parity.
We check the odd or even by using bitwise AND operator. If (b & 1) is true means odd else even.
C++ program to find parity of a number efficiently
So, here is the C++ implementation of the above algorithm:-
Output:-
Thanks for reading this tutorial. I hope it helps you !!
Leave a Reply
- Trending Categories
- Selected Reading
2d Parity Check Program Compatibility
In this article, we will be discussing a program to find the parity of a given number N.
Parity is defined as the number of set bits (number of ‘1’s) in the binary representation of a number.
If the number of ‘1’s in the binary representation are even, the parity is called even parity and if the number of ‘1’s in the binary representation is odd, the parity is called odd parity.
If the given number is N, we can perform the following operations.
- y = N ^ (N >> 1)
- y = y ^ (y >> 2)
- y = y ^ (y >> 4)
- y = y ^ (y >> 8)
- y = y ^ (y >> 16)
Once all these operations are done, the rightmost bit in y will represent the parity of the number. If the bit is 1, the parity would be odd and if the bit would be 0, the parity will be even.
Example
2d Parity Check Program Cal
Output
2d Parity Check Program Can
- Related Questions & Answers